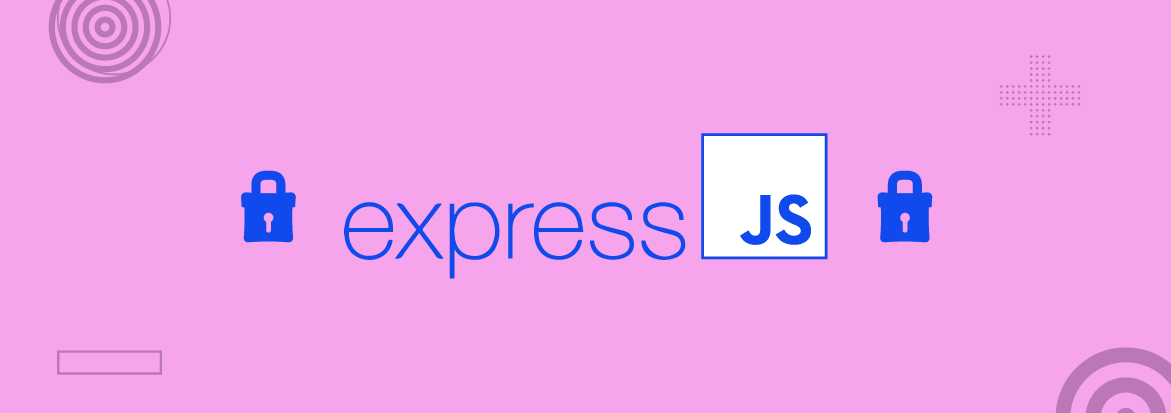
If you’ve ever delved into Node.js, then there’s a high chance that you’ll also encounter Express.js — a minimalist web framework built to create Node.js web applications. It comes with HTTP utilities for rapidly developing APIs and provides the foundations of other major and popular Node.js frameworks such as Feathers, LoopBack, Blueprint, and Nest.js.
While Express is generally robust for assisting your full stack application builds and prototypes, it is not perfect when it comes to security. The reason for this is because Express is designed for creating web applications — not securing your Node.js server from vulnerabilities. The defaults leave the HTTP headers mostly open, in part, to assist with the rapid application development. This is where Helmet.js steps in.
Helmet.js fills in the gap between Node.js and Express.js by securing HTTP headers that are returned by your Express apps. HTTP, by design, is open in nature and highly insecure. It can leak sensitive information about your app and leave your data open to anyone with some tech skills to see.
In short, using the preset default HTTP headers is a sure way to quickly get your app and running, but at the potential cost of anyone having access to it in a malicious way. What makes it worse is that because end users tend to be uneducated over the importance of HTTPS over HTTP, developers tend to ignore it unless otherwise forced to use HTTPS by their security policies.
In this article, we will go over how to install Helmet.js, what the different headers are, and how to use them in Helmet.js.
How to install Helmet.js for Express.js
To install Helmet.js for your Express.js application, use the following sequence of commands.
First, start by installing helmet.
Now, include it into your app like this:
That’s basically it. Now you’re ready to begin configuring your API’s headers for extra added security.
How Helmet.js works and how to use it
Helmet.js comes with a collection of Node modules that you can use to interface to Express to increase the HTTP header security. How does it work? It lets you configure the headers and prevent common vulnerabilities such as clickjacking, implementation of strict HTTP, and download options for vulnerable browsers such as IE8.
When you use Helmet.js, you can also configure Content-Security-Policy to force subsequent developers working on public-facing APIs that require HTTP to approach the code with a security-first mindset.
Here is a list of HTTP headers supported by Helmet.js and how to use them.
Content-Security-Policy
helmet.contentSecurityPolicy(options) lets you set the Content-Security-Policy which allows you to mitigate cross-site scripting attacks. If no directive is applied by the developer, the following policy is set as the default:
Here is an example of the module in use:
useDefaults applies all the defaults as stated above and overrides it with the supplied directives directly below.
But what is a Content-Security-Policy — or CSP?
A CSP lets the browser know how to process certain directions and minimize cross-site scripting vulnerabilities. When CSP isn’t set on your headers, the browser is set to accept all HTTP responses by default. This includes resources from external domains.
By default, Helmet.js doesn’t add CSP as part of its default configuration as it can block things like CDN file inclusion — which can negatively impact a user’s experience. While a blanket exclusion of external loading of scripts is not recommended, you can create a whitelist of domains that is monitored and maintained by the development team.
Expect-CT
helmet.expectCT sets the Expect-CT header. This prevents mis-issued SSL certificates. There are three parameters that you can use.
- maxAge – determines the number of sections to expect Certificate Transparency.
- enforce – if true, the user agent should refuse future connections that violate the Certificate Transparency policy. If not set, it defaults to false.
- reportUri – if anything fails, it will report the failure to the URL supplied.
Here is an example of the module in use:
X-DNS-Prefetch-Control
helmet.dnsPrefetchControl lets you set the X-DNS-Prefetch-Control header in Express. This helps control DNS prefetching and improves user privacy.
Here’s how to use it:
X-Frame-Options
helmet.frameguard sets the X-Frame-Options in the header to prevent clickjacking attacks.
Here is how you can use it:
action takes either deny or sameorigin. By default, Helmet sets this to sameorigin.
X-Powered-By
helmet.hidePoweredBy removes the X-Powered-By broswer, which can give valuable information to malicious users to exploit. In Express, this information is sent to the public by default.
Here is how you can use it:
Strict-Transport-Security
helmet.hsts sets the Strict-Transport-Security header. This tells the browser to prefer HTTPS over HTTP. The maxAge parameter lets the number of seconds browsers should remember to prefer HTTPS. By default, this figure is 15552000 — or 180 days.
You can also include subdomains as well via includeSubDomains. Here is how to use it:
X-Download-Options
helmet.isNoOpen sets the X-Download-Options header. This is specific to the vulnerabilities in IE 8 and forces potentially unsafe downloads to be saved and prevents the execution of HTML in your site’s context.
Here is how to use it:
X-Content-Type-Options
helmet.noSniff sets the X-Content-Type-Options head to nosniff. This prevents MIME type sniffing.
Here is how to use it:
Referrer-Policy
helmet.referrerPolicy sets the Referrer-Policy header. This controls the information inside the Referer header.
Here is an example of how to use it:
By default, Helmet.js sets this to no-referrer.
X-XSS-Protection
helmet.xssFilter prevents cross-site scripting. While browsers come with a filter that prevents this by default, it is not evenly applied and bugginess can range depending on if the end-user is using Chrome, IE, Firefox, Safari, or something else.
Using helmet.xssFilter puts another layer of security on your API. Here is how to use it:
Conclusion
HTTP headers are something that is often ignored by developers. Most of the time, it’s because we are too preoccupied with trying to make our APIs work and behave the way we want them to.
HTTP headers are generally an afterthought. This can lead to vulnerabilities and sharing of data that can be easily used to exploit your application. It is not that hard to view HTTP headers in the browser. A user simply needs to right-click, navigate to dev tools, then over to the Network tab and check the headers on a file that was fetched from the server. This will let a malicious user see what kind of techniques they can use to exploit your APIs.
By design, HTTP headers are available for all to see. The browser uses this information to prevent certain things from happening to your site — such as cross-domain hijacking and enforcing the usage of HTTPS.
Using Helmet.js with your Express application is a quick and simple way to create a layer of security by switching from Express defaults to a more secure set of defaults. In addition to this, Helmet.js also lets you configure your HTTP headers with ease through the available modules.